How do we determine the length of a string in C++? This question is a common one among both novice programmers and seasoned developers. Strings in C++ are pivotal in managing and manipulating text, making it crucial to understand how to measure their length accurately. Understanding the length of a string helps in efficiently allocating memory, optimizing performance, and ensuring the integrity of data processing tasks. In this comprehensive guide, we'll delve into the various methods available in C++ for calculating string length, exploring the nuances and best practices associated with each approach.
Strings are fundamental to any programming language, and C++ is no exception. While C++ offers a robust set of tools and libraries for handling strings, the task of determining their length can present challenges if not approached correctly. From the standard library's std::string
to character arrays and pointers, each method of string representation in C++ offers unique advantages and disadvantages. By examining these different approaches, we'll provide you with a thorough understanding of how to measure string length effectively in C++.
This article aims to empower you with the knowledge needed to navigate the complexities of string manipulation in C++. We'll cover the theoretical aspects, practical implementations, and common pitfalls associated with measuring string length. Whether you're a student learning C++ or a professional seeking to refine your coding skills, this guide will serve as an invaluable resource. Let's dive into the world of C++ strings and discover the most efficient ways to determine their length.
Table of Contents
- History and Evolution of Strings in C++
- Understanding C++ String Representation
- Measuring String Length Using std::string
- Character Arrays and Pointers
- Using the strlen() Function
- Handling Wide Strings
- String Length in Custom Data Types
- Performance Considerations
- Common Pitfalls and How to Avoid Them
- Practical Examples and Code Snippets
- Advanced String Length Manipulation Techniques
- Optimizing Memory Usage with String Length
- Real-World Applications
- Frequently Asked Questions
- Conclusion
History and Evolution of Strings in C++
The concept of strings in C++ has evolved significantly since the language's inception. In the early days of C, strings were represented as arrays of characters terminated by a null character. This method, while effective, posed several challenges in terms of memory management and error handling. With the introduction of C++, a more sophisticated and robust approach to string handling was developed, leveraging the power of object-oriented programming.
The Standard Template Library (STL) brought about a major shift in how strings were handled in C++. The introduction of the std::string
class provided developers with a more intuitive and flexible way to work with strings. This class encapsulated the complexities of memory management and offered a range of member functions for string manipulation. The evolution of strings in C++ has been driven by the need for efficiency, reliability, and ease of use, culminating in the modern string handling capabilities we see today.
As C++ continued to develop, further enhancements were made to the string class to improve performance and functionality. These improvements included optimizations for copy operations, better support for internationalization through wide strings, and the introduction of the std::wstring
class for handling wide character sets. The evolution of strings in C++ reflects the language's commitment to balancing power and simplicity, making it a popular choice for developers worldwide.
Understanding C++ String Representation
In C++, strings can be represented in various ways, each with its own set of characteristics and use cases. The two primary representations are character arrays and the std::string
class. Character arrays are essentially sequences of characters stored in contiguous memory locations, terminated by a null character to indicate the end of the string. This representation is directly inherited from C and offers fine-grained control over memory usage.
The std::string
class, on the other hand, is a part of the C++ Standard Library and provides a more abstracted and user-friendly interface for string manipulation. It manages memory automatically, eliminating the risk of buffer overflows and other common errors associated with character arrays. The std::string
class also offers a wide range of member functions for performing operations such as concatenation, comparison, and substring extraction.
In addition to these two primary representations, C++ also supports wide strings through the std::wstring
class. Wide strings are used for handling characters that require more than one byte of storage, such as those in Unicode. This representation is particularly useful for applications that need to support internationalization and work with diverse character sets. Understanding the different string representations in C++ is essential for selecting the appropriate approach for a given application.
Measuring String Length Using std::string
One of the most straightforward ways to determine the length of a string in C++ is by using the std::string
class. This class provides a member function called length()
that returns the number of characters in the string. The length()
function is simple to use and offers a reliable method for measuring string length without the risk of errors associated with manual calculations.
To use the length()
function, simply create an instance of the std::string
class and call the function on that instance. For example:
#include #include int main() { std::string myString ="Hello, World!"; std::cout << "The length of the string is: " << myString.length() << std::endl; return 0; }
This code snippet demonstrates how to create a string and measure its length using the length()
function. The result is then printed to the console. The std::string
class also provides a size()
function, which serves the same purpose as length()
. Both functions are interchangeable and return the same result.
In addition to the length()
function, the std::string
class offers other useful member functions for string manipulation, such as empty()
, clear()
, and resize()
. These functions provide developers with a comprehensive toolkit for working with strings in C++.
Character Arrays and Pointers
Character arrays are a traditional way of representing strings in C and C++. They offer a lower-level approach to string handling, providing developers with direct control over memory allocation and manipulation. Character arrays are essentially sequences of characters stored in contiguous memory locations, with a null character ('\0') indicating the end of the string.
When using character arrays, it's important to ensure that the array is properly terminated with a null character, as this is how functions like strlen()
determine the length of the string. For example:
#include #include int main() { char myString[] ="Hello, World!"; std::cout << "The length of the string is: " << strlen(myString) << std::endl; return 0; }
In this example, the strlen()
function is used to calculate the length of the character array. This function counts the number of characters until it encounters the null character, providing the length of the string. It's important to note that strlen()
does not include the null character in its count.
While character arrays offer greater control over memory management, they also come with risks, such as buffer overflows and memory leaks. Careful attention must be paid to ensure that arrays are properly sized and terminated to avoid these issues. Despite these challenges, character arrays remain a valuable tool for developers who require fine-grained control over string handling.
Using the strlen() Function
The strlen()
function is a standard library function in C and C++ that calculates the length of a null-terminated character array. It counts the number of characters in the array until it encounters the null character, providing an accurate measure of the string's length. The strlen()
function is commonly used with character arrays, but it can also be applied to strings represented as pointers.
To use the strlen()
function, simply pass the character array or pointer to the function as an argument. For example:
#include #include int main() { const char* myString ="Hello, World!"; std::cout << "The length of the string is: " << strlen(myString) << std::endl; return 0; }
In this example, a pointer to a string literal is passed to the strlen()
function, which calculates and returns the length of the string. The strlen()
function is efficient and widely used, but it requires that the input string be properly null-terminated. Any deviation from this requirement can result in undefined behavior.
It's important to note that strlen()
operates in O(n) time complexity, where n is the length of the string. This means that for very large strings, the performance of strlen()
may become a consideration. However, for most practical applications, the function provides a reliable and efficient means of calculating string length.
Handling Wide Strings
In addition to traditional strings, C++ supports wide strings through the std::wstring
class. Wide strings are used to represent characters that require more than one byte of storage, such as those in Unicode. This representation is particularly useful for applications that need to support multiple languages and character sets.
Wide strings are similar to regular strings but use the wchar_t
data type instead of char
. The std::wstring
class provides similar member functions to std::string
, including length()
and size()
, for measuring string length. For example:
#include #include int main() { std::wstring myWideString = L"Hello, World!"; std::wcout << L"The length of the wide string is: " << myWideString.length() << std::endl; return 0; }
In this code snippet, a wide string is created using the std::wstring
class, and its length is measured using the length()
function. The L
prefix is used to denote a wide string literal, and std::wcout
is used for outputting wide characters.
Handling wide strings requires careful consideration of character encoding and compatibility. Wide strings are typically used in conjunction with Unicode or other multi-byte character encodings to ensure that characters are represented accurately across different platforms and locales. Understanding how to work with wide strings is essential for developing applications that require robust internationalization support.
String Length in Custom Data Types
In some cases, developers may need to define custom data types that include string representations. This can be useful for encapsulating additional data or implementing specialized functionality. When working with custom data types, it's important to consider how string length will be measured and stored within the type.
Custom data types can be implemented as classes or structures that include one or more string members. These members can be represented using std::string
, character arrays, or other string types. When defining a custom data type, consider adding member functions or operators for calculating and accessing string length. For example:
#include #include class CustomString { private: std::string str; public: CustomString(const std::string& s) : str(s) {} size_t length() const { return str.length(); } }; int main() { CustomString myString("Hello, World!"); std::cout << "The length of the custom string is: " << myString.length() << std::endl; return 0; }
In this example, a custom data type CustomString
is defined with a member function length()
that returns the length of the encapsulated string. This approach allows developers to encapsulate string handling functionality within a custom type, providing a clean and organized interface for working with strings.
When designing custom data types, it's important to consider the implications of string length on memory usage and performance. Ensure that string operations are efficient and that the data type's interface is intuitive and consistent with standard string handling practices in C++.
Performance Considerations
When working with strings in C++, performance is a key consideration. The choice of string representation and the methods used to measure string length can have a significant impact on the efficiency of an application. Understanding the performance characteristics of different string handling techniques is essential for optimizing both speed and memory usage.
Using the std::string
class is generally recommended for most applications due to its ease of use and built-in memory management. The length()
and size()
functions of std::string
are efficient and provide constant-time access to the string's length. This makes them ideal for applications where string length is frequently accessed or modified.
However, there are scenarios where character arrays or pointers may be more appropriate, particularly when fine-grained control over memory is required. In such cases, the strlen()
function offers a reliable means of measuring string length, but developers should be mindful of its linear time complexity and the need for proper null termination.
Wide strings, represented by the std::wstring
class, introduce additional considerations related to character encoding and memory usage. While they offer robust support for internationalization, wide strings may consume more memory and require careful management to ensure compatibility across different platforms.
Overall, the choice of string representation and length measurement method should be guided by the specific requirements of the application, including factors such as performance, memory usage, and ease of maintenance.
Common Pitfalls and How to Avoid Them
Working with strings in C++ can present various challenges and pitfalls that developers need to be aware of. Understanding these common issues and knowing how to avoid them is crucial for ensuring the reliability and robustness of your code.
1. Buffer Overflows
One of the most common pitfalls when working with character arrays is buffer overflows. This occurs when a string exceeds the allocated memory space, potentially leading to undefined behavior and security vulnerabilities. To avoid buffer overflows, ensure that character arrays are properly sized and that all string operations are bounds-checked.
2. Null Termination
Strings represented as character arrays must be properly null-terminated with a '\0' character. Failing to null-terminate a string can result in erroneous length calculations and undefined behavior. Always verify that strings are null-terminated, especially when performing manual memory management or interfacing with C-style APIs.
3. Memory Leaks
Memory leaks occur when dynamically allocated memory is not properly deallocated, leading to wasted resources and potential application crashes. When using new
and delete
for string allocation, ensure that every allocation is matched with a corresponding deallocation. Consider using smart pointers or the std::string
class to manage memory automatically.
4. Incorrect Usage of strlen()
The strlen()
function is designed for null-terminated strings. Using strlen()
on non-null-terminated strings or uninitialized memory can result in erroneous length calculations and undefined behavior. Always ensure that strings are properly initialized and null-terminated before passing them to strlen()
.
By being aware of these common pitfalls and implementing best practices for string handling, developers can avoid many of the errors and issues associated with string manipulation in C++.
Practical Examples and Code Snippets
To reinforce the concepts covered in this article, let's explore some practical examples and code snippets that demonstrate different methods for measuring string length in C++.
Example 1: Using std::string
#include #include int main() { std::string myString ="C++ Programming"; std::cout << "The length of the string is: " << myString.length() << std::endl; return 0; }
This example demonstrates how to create a std::string
and measure its length using the length()
function. The result is printed to the console.
Example 2: Using Character Arrays
#include #include int main() { char myString[] ="Hello, World!"; std::cout << "The length of the string is: " << strlen(myString) << std::endl; return 0; }
This example shows how to measure the length of a character array using the strlen()
function. The length is calculated and displayed to the console.
Example 3: Using std::wstring
#include #include int main() { std::wstring myWideString = L"Wide String Example"; std::wcout << L"The length of the wide string is: " << myWideString.length() << std::endl; return 0; }
This example illustrates how to work with wide strings using the std::wstring
class. The length of the wide string is measured and outputted using std::wcout
.
These examples provide a practical introduction to measuring string length in C++ using different string representations. By experimenting with these code snippets, you can gain a deeper understanding of string handling techniques and their applications.
Advanced String Length Manipulation Techniques
In addition to the basic methods for measuring string length discussed earlier, C++ offers several advanced techniques for manipulating and analyzing string length. These techniques are useful for developers who need to perform complex string operations or optimize performance in specific scenarios.
1. Substring Extraction
Substring extraction involves obtaining a portion of a string based on specified start and end positions. The std::string
class provides the substr()
function for this purpose. By extracting substrings, developers can manipulate and analyze specific segments of a string.
#include #include int main() { std::string myString ="Advanced C++ Techniques"; std::string subString = myString.substr(9, 3); // Extracts "C++" std::cout << "Substring: " << subString << std::endl; return 0; }
This example demonstrates how to extract a substring from a string using the substr()
function. The resulting substring is printed to the console.
2. Regular Expressions
Regular expressions provide a powerful way to search, match, and manipulate strings based on complex patterns. The C++ Standard Library includes the
header, which contains classes and functions for working with regular expressions.
#include #include #include int main() { std::string text ="The quick brown fox jumps over the lazy dog"; std::regex pattern("\\b\\w{5}\\b"); // Match words with exactly 5 letters std::sregex_iterator it(text.begin(), text.end(), pattern); std::sregex_iterator end; while (it != end) { std::cout << "Matched word: " << it->str() << std::endl; ++it; } return 0; }
This example demonstrates how to use regular expressions to find words with exactly five letters in a given string. Regular expressions offer a flexible and efficient way to perform complex string operations.
3. Custom String Algorithms
Developers can also implement custom algorithms to perform specialized string operations, such as calculating the frequency of character occurrences or detecting palindromes. These algorithms can be tailored to meet specific application requirements and optimize performance.
#include #include #include void calculateFrequency(const std::string& str) { std::unordered_map frequencyMap; for (char ch : str) { frequencyMap[ch]++; } for (const auto& pair : frequencyMap) { std::cout << pair.first << ": " << pair.second << std::endl; } } int main() { std::string myString ="frequency"; calculateFrequency(myString); return 0; }
This example shows how to implement a custom algorithm to calculate the frequency of each character in a string. Custom algorithms provide developers with the flexibility to tailor string operations to their specific needs.
By exploring these advanced techniques, developers can enhance their ability to manipulate and analyze strings in C++, enabling them to tackle complex problems and optimize performance in their applications.
Optimizing Memory Usage with String Length
Memory usage is a critical consideration when working with strings in C++, especially in resource-constrained environments or applications that handle large volumes of data. By optimizing memory usage, developers can improve application performance and reduce the risk of memory-related issues.
1. Efficient Memory Allocation
When using character arrays, it's important to allocate memory efficiently to minimize waste and avoid buffer overflows. Consider using dynamic memory allocation with new
and delete
to allocate only the necessary amount of memory for a string. For example:
#include int main() { const char* originalString ="Optimize Memory Usage"; size_t length = strlen(originalString); char* dynamicString = new char[length + 1]; // Allocate memory for the string strcpy(dynamicString, originalString); // Copy the string std::cout << "Dynamic string: " << dynamicString << std::endl; delete[] dynamicString; // Deallocate memory return 0; }
This example demonstrates how to use dynamic memory allocation to create a character array of the exact size needed to store a string. By managing memory efficiently, developers can optimize both performance and resource usage.
2. Avoiding Unnecessary Copies
String operations that involve copying or concatenating strings can lead to increased memory usage and reduced performance. To avoid unnecessary copies, consider using references or pointers where possible, and take advantage of move semantics to transfer ownership of resources without copying.
3. Leveraging std::string
Capacity
The std::string
class provides a capacity()
function that returns the total number of characters that the string can hold without reallocating memory. By pre-allocating sufficient capacity using the reserve()
function, developers can minimize memory reallocations and improve performance during string modifications.
#include #include int main() { std::string myString; myString.reserve(100); // Pre-allocate memory for 100 characters myString ="Optimized String"; std::cout << "String capacity: " << myString.capacity() << std::endl; return 0; }
This example demonstrates how to use the reserve()
function to pre-allocate memory for a std::string
. By optimizing capacity, developers can reduce the overhead associated with frequent memory reallocations.
By implementing these strategies, developers can optimize memory usage in their C++ applications, ensuring efficient performance and reducing the risk of memory-related issues.
Real-World Applications
The ability to accurately measure and manipulate string length in C++ is essential for a wide range of real-world applications. From text processing and data analysis to user interfaces and network communication, strings play a vital role in software development. Understanding how to work with strings effectively can unlock new possibilities and enhance the capabilities of your applications.
1. Natural Language Processing (NLP)
In the field of natural language processing, strings are used to represent and analyze human language data. Accurate string length measurement is crucial for tasks such as tokenization, stemming, and sentiment analysis. By leveraging C++ string handling capabilities, developers can build efficient NLP applications that process large volumes of text data.
2. User Interfaces
User interfaces often involve the display and manipulation of text, making string length a key consideration. Whether designing a graphical user interface (GUI) or a command-line application, developers must ensure that strings are properly sized and displayed. C++ provides the tools needed to manage string length effectively, enabling the creation of intuitive and responsive user interfaces.
3. File and Data Processing
String manipulation is a fundamental aspect of file and data processing applications. Whether parsing CSV files or processing JSON data, developers must accurately measure and manipulate string length to extract and analyze information. C++ offers a range of string handling techniques that can be applied to various data processing tasks.
4. Network Communication
In network communication applications, strings are used to represent data transmitted between devices. Accurate string length measurement is essential for encoding and decoding messages, ensuring data integrity, and optimizing network performance. By utilizing C++ string handling capabilities, developers can build reliable and efficient network communication systems.
These real-world applications highlight the importance of understanding string length in C++ and demonstrate the diverse ways in which strings are used across different domains. By mastering string handling techniques, developers can create powerful and versatile applications that meet the needs of modern users.
Frequently Asked Questions
1. What is the most efficient way to measure string length in C++?
The most efficient way to measure string length in C++ is by using the length()
or size()
member functions of the std::string
class. These functions provide constant-time access to the string's length and are recommended for most applications.
2. Can I use strlen()
with std::string
?
No, strlen()
is designed for null-terminated character arrays and should not be used with std::string
. Instead, use the length()
or size()
member functions provided by the std::string
class.
3. How do I handle strings with special characters or Unicode?
To handle strings with special characters or Unicode, consider using the std::wstring
class, which supports wide characters. Additionally, libraries such as ICU (International Components for Unicode) provide extensive support for Unicode and internationalization.
4. What are the performance implications of using std::wstring
?
While std::wstring
provides robust support for wide characters, it may consume more memory than std::string
due to the increased size of wchar_t
. Developers should consider these performance implications, especially in memory-constrained environments.
5. How do I prevent buffer overflows when using character arrays?
To prevent buffer overflows, ensure that character arrays are properly sized and null-terminated. Use functions like strncpy()
or snprintf()
to perform bounds-checked string operations, and consider using std::string
for automatic memory management.
6. Can I use C++ string handling techniques in other programming languages?
While C++ string handling techniques are specific to the language, many concepts are applicable to other programming languages. For example, the importance of memory management, null termination, and efficient string manipulation is universal across programming languages.
Conclusion
Understanding the length of a string in C++ is a fundamental aspect of string manipulation and text processing. By exploring the various methods for measuring string length, including std::string
, character arrays, and wide strings, developers can gain a comprehensive understanding of string handling in C++. This knowledge empowers developers to build robust, efficient, and versatile applications that leverage the full potential of C++ string capabilities.
Throughout this article, we've covered the theoretical and practical aspects of string length measurement, explored advanced techniques, and addressed common pitfalls. By mastering these concepts, you'll be well-equipped to tackle a wide range of string-related challenges in your C++ projects. As you continue to develop your skills, remember to stay informed about the latest advancements in C++ and string handling to ensure that your applications remain cutting-edge and efficient.
For further reading and exploration, consider visiting [cplusplus.com](http://www.cplusplus.com), a valuable resource for C++ documentation and tutorials. By continuing to learn and experiment with C++ string handling, you'll be able to unlock new possibilities and achieve your programming goals.

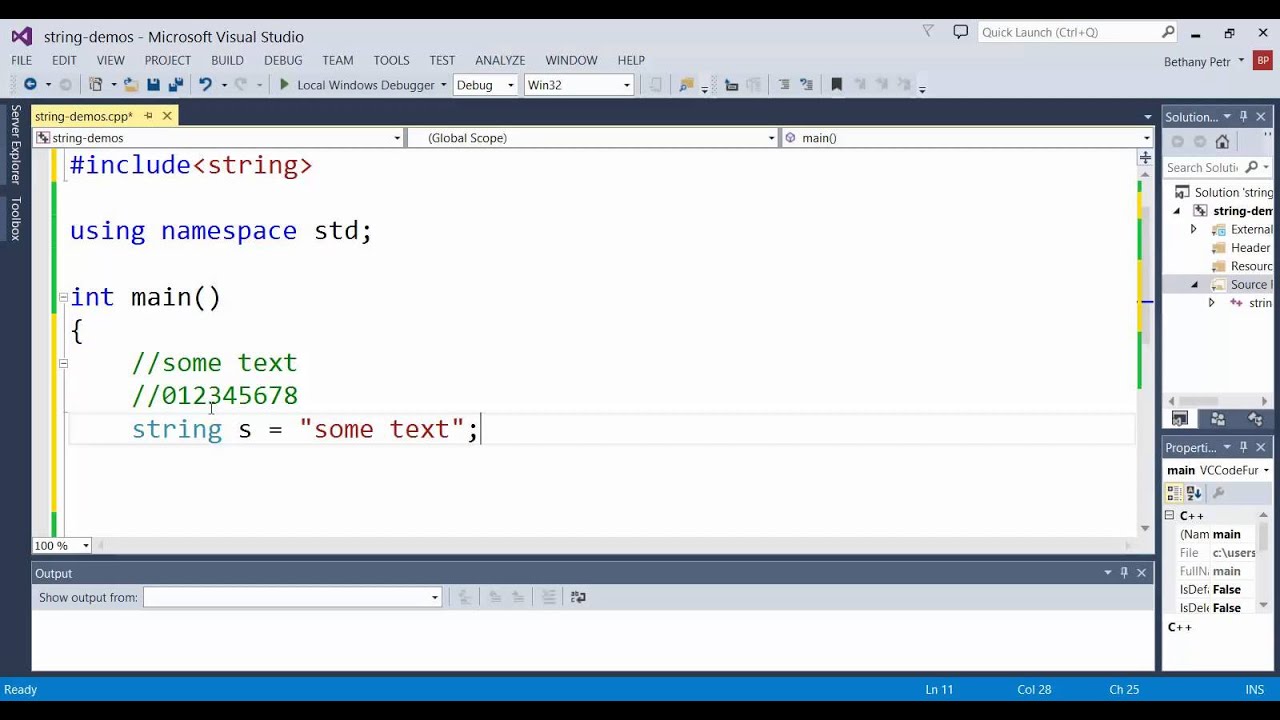